Context
Compare the contents of two collections of strings (no nested objects). Returns the string true
if there is a common item, or false
if there is not.
Level 1 - Simple
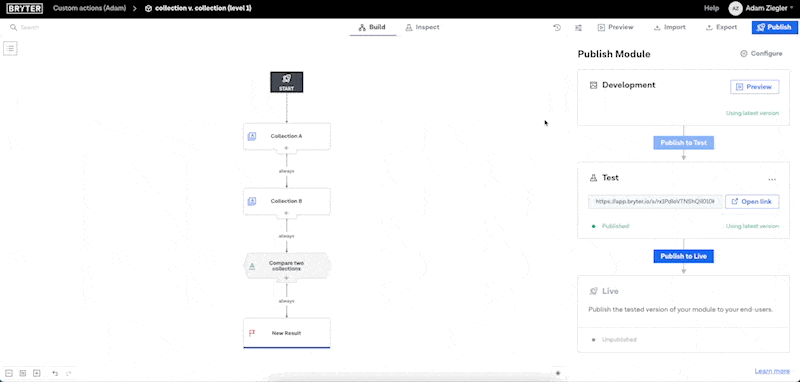
Level 2 - Not as Simple
The collections can originate from a filtered query of a database, but you have to first flatten that collection by performing a collection operation to convert it to string and then using another custom action - the split string action - to convert into a collection of strings. Then you can compare the collections.
For example, if you have a database of countries with assigned risk levels, and you want to compare a user-provided list of countries (e.g. the countries where user does business) to the collection of high risk countries, this method will work.

Metadata
{
"key": "compare-collections",
"name": "Compare two collections",
"description": "Look for Common Items in Two Collections",
"developerEmail": "email@bryter.io",
"parameters": [
{
"name": "collection A",
"description": "The first collection to compare",
"type": {
"type": "collection",
"items": "text"
}
},
{
"name": "collection B",
"description": "The second collection to compare",
"type": {
"type": "collection",
"items": "text"
}
}
],
"result": {
"type": "text",
"description": "The true or false result of the comparison"
}
}
Function
function main(collectionA: string[], collectionB: string[]): string {
// compare two arrays and return true if they have any common items
const result = collectionA.some((item) => collectionB.includes(item));
// convert the boolean result to a string
return result.toString();
}